Because I have a putty terminal running on my PC most every day (mostly sitting there doing nothing, waiting for a command,) I thought I’d put that open terminal to use and have it display stock tickers. So I asked ChatGPT to create a bash shell script that display a ticker and it’s price from the internet. I am not a good programmer/developer, and I can barely write a few scripts, but by using ChatGPT I was able to produce what I wanted in a short amount of time. Below is the script that it produced:
#!/usr/bin/env bash set -e LANG=C LC_NUMERIC=C SYMBOLS=("$@") if ! $(type jq > /dev/null 2>&1); then echo "'jq' is not in the PATH. (See: https://stedolan.github.io/jq/)" exit 1 fi if [ -z "$SYMBOLS" ]; then echo "Usage: ./ticker.sh AAPL MSFT GOOG BTC-USD" exit fi FIELDS=(symbol marketState regularMarketPrice regularMarketChange regularMarketChangePercent \ preMarketPrice preMarketChange preMarketChangePercent postMarketPrice postMarketChange postMarketChangePercent) API_ENDPOINT="https://query1.finance.yahoo.com/v6/finance/quote?lang=en-US®ion=US&corsDomain=finance.yahoo.com" if [ -z "$NO_COLOR" ]; then : "${COLOR_BOLD:=\e[1;37m}" : "${COLOR_GREEN:=\e[32m}" : "${COLOR_RED:=\e[31m}" : "${COLOR_RESET:=\e[00m}" fi symbols=$(IFS=,; echo "${SYMBOLS[*]}") fields=$(IFS=,; echo "${FIELDS[*]}") results=$(curl --silent "$API_ENDPOINT&fields=$fields&symbols=$symbols" \ | jq '.quoteResponse .result') query () { echo $results | jq -r ".[] | select(.symbol == \"$1\") | .$2" } for symbol in $(IFS=' '; echo "${SYMBOLS[*]}" | tr '[:lower:]' '[:upper:]'); do marketState="$(query $symbol 'marketState')" if [ -z $marketState ]; then printf 'No results for symbol "%s"\n' $symbol continue fi preMarketChange="$(query $symbol 'preMarketChange')" postMarketChange="$(query $symbol 'postMarketChange')" if [ $marketState == "PRE" ] \ && [ $preMarketChange != "0" ] \ && [ $preMarketChange != "null" ]; then nonRegularMarketSign='*' price=$(query $symbol 'preMarketPrice') diff=$preMarketChange percent=$(query $symbol 'preMarketChangePercent') elif [ $marketState != "REGULAR" ] \ && [ $postMarketChange != "0" ] \ && [ $postMarketChange != "null" ]; then nonRegularMarketSign='*' price=$(query $symbol 'postMarketPrice') diff=$postMarketChange percent=$(query $symbol 'postMarketChangePercent') else nonRegularMarketSign='' price=$(query $symbol 'regularMarketPrice') diff=$(query $symbol 'regularMarketChange') percent=$(query $symbol 'regularMarketChangePercent') fi if [ "$diff" == "0" ] || [ "$diff" == "0.0" ]; then color= elif ( echo "$diff" | grep -q ^- ); then color=$COLOR_RED else color=$COLOR_GREEN fi if [ "$price" != "null" ]; then printf "%-10s$COLOR_BOLD%8.2f$COLOR_RESET" $symbol $price printf "$color%10.2f%12s$COLOR_RESET" $diff $(printf "(%.2f%%)" $percent) printf " %s\n" "$nonRegularMarketSign" fi done
This produced the following, for example:

This works well, however I want the script to display a number of different tickers and have the data refresh every 10 seconds. The next script produced is the following ticker_loop.sh. I added my tickers
#!/bin/bash while true; do clear ./ticker.sh PLTR SOFI HIMS ARKK AHT QYLD MSFT ONON sleep 10 done
This is what I was looking for! So I simply had to modify both to run as executible / binary files. To do so, use the command:
chmod +x ticker_loop.sh
Lastly, run the script with the command ./ticker_loop.sh
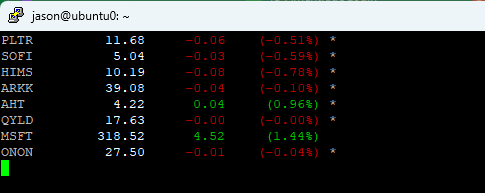